Controller and its Basic Decorators in NestJS

- Published on
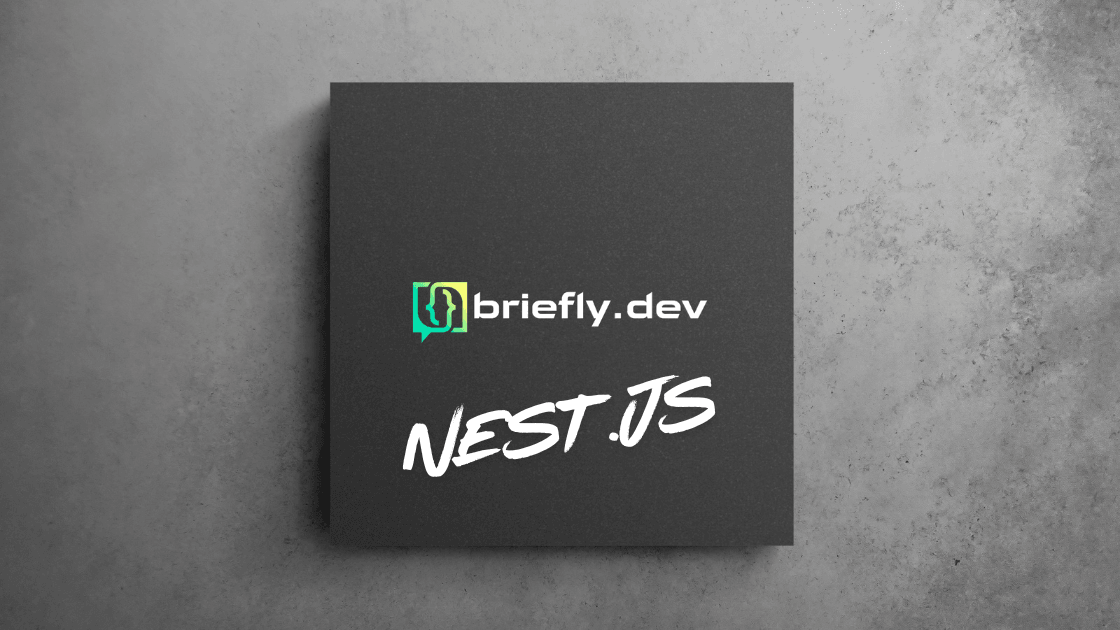
Controllers in NestJS
A controller in NestJS is responsible for handling incoming requests and returning responses to the client. Each controller is associated with a specific route and can handle multiple HTTP methods, such as GET, POST, PUT, PATCH, and DELETE.
Here's a basic example of a controller in NestJS:
import { Controller, Get } from '@nestjs/common'
@Controller('users')
export class UsersController {
@Get()
findAll(): string {
return 'This action returns all users'
}
}
In this example, the UsersController handles requests to the /users route, and the @Get() decorator defines a route for the HTTP GET method.
Common Decorators in NestJS
NestJS provides a variety of decorators to manage routes, parameters, and responses. Let's take a closer look at some of the most commonly used ones.
@Get(), @Post(), @Put(), @Patch(), and @Delete()
These decorators correspond to the HTTP methods GET, POST, PUT, PATCH, and DELETE, respectively.
import { Controller, Post, Put, Patch, Delete, Get } from '@nestjs/common'
@Controller('products')
export class ProductsController {
@Get()
findAll(): string {
return 'Get all products'
}
@Post()
create(): string {
return 'Create a product'
}
@Put(':id')
update(@Param('id') id: string): string {
return `Update product with ID ${id}`
}
@Patch(':id')
partialUpdate(@Param('id') id: string): string {
return `Partially update product with ID ${id}`
}
@Delete(':id')
remove(@Param('id') id: string): string {
return `Delete product with ID ${id}`
}
}
@Body(), @Query(), @Param(), @Headers()
These decorators allow you to easily access request data, such as the body, query parameters, route parameters, and headers.
import { Controller, Post, Body, Query, Param, Headers } from '@nestjs/common'
@Controller('orders')
export class OrdersController {
@Post()
createOrder(
@Body() body: any,
@Query('discount') discount: string,
@Param('id') id: string,
@Headers('authorization') authHeader: string
): string {
console.log(body)
console.log(`Discount: ${discount}`)
console.log(`Order ID: ${id}`)
console.log(`Authorization Header: ${authHeader}`)
return 'Order created'
}
}
@HttpCode() and @Redirect()
- @HttpCode(): This decorator allows you to specify the HTTP status code of the response.
- @Redirect(): This decorator is used to redirect the client to another URL.
import { Controller, Get, HttpCode, Redirect } from '@nestjs/common'
@Controller('docs')
export class DocsController {
@Get()
@HttpCode(200)
getDocumentation(): string {
return 'Documentation content'
}
@Get('google')
@Redirect('https://www.google.com', 302)
redirectToGoogle() {}
}
In the example above, getDocumentation() will return a response with a 200 HTTP status code, while redirectToGoogle() will redirect the client to Google with a 302 status code.