Creating a Custom Decorator in NestJS
By Łukasz Kallas

- Published on
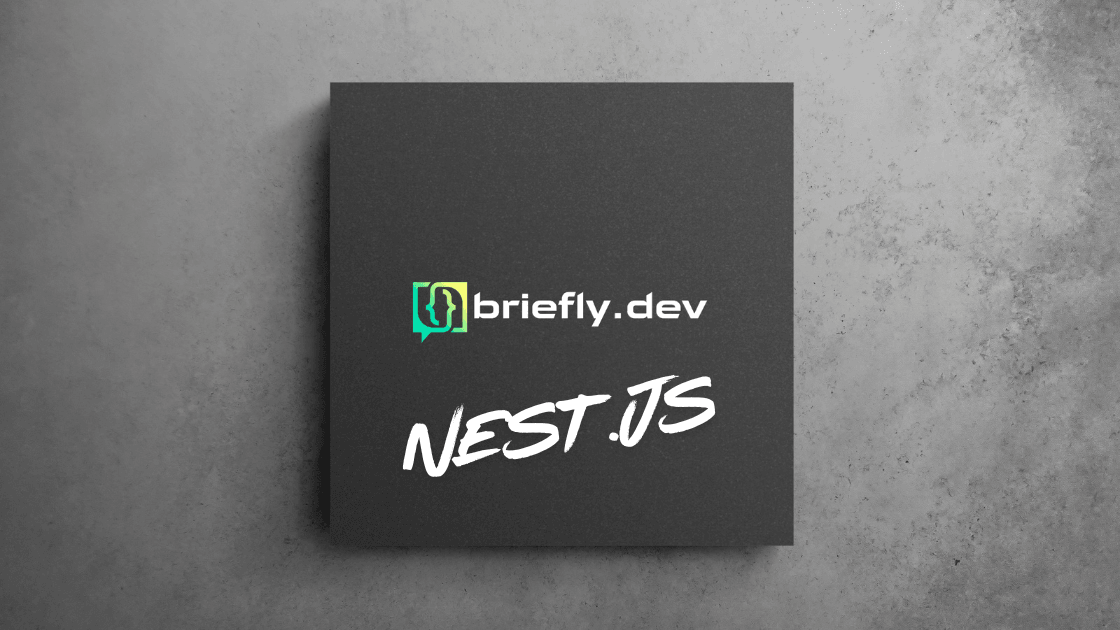
Sharing
Today we'll explore how to create a custom decorator in a NestJS project. Custom decorators are useful to simplify logic and make your code cleaner and more reusable.
What is a Decorator?
A decorator in NestJS is a special function that can be applied to classes, methods, or properties. It allows you to modify behaviors, add metadata, or apply reusable logic in an expressive and declarative way.
Step-by-Step Guide to Creating a Custom Decorator
Step 1: Create a Decorator Logic
In decorators/time.decorator.ts
lets create a decorator, which will log the controller execution time onto console:
export function Time(): MethodDecorator {
return (target: any, key: string | symbol, descriptor: PropertyDescriptor) => {
const originalMethod = descriptor.value
descriptor.value = function (...args: any[]) {
console.time(key.toString())
const result = originalMethod.apply(this, args)
console.timeEnd(key.toString())
return result
}
return descriptor
}
}
Step 2: Apply Decorator on a Route
import { Time } from './decorators/time.decorator'
@Controller()
export class AppController {
constructor(private readonly appService: AppService) {}
@Get()
@Time()
getHello(): string {
return this.appService.getHello()
}
}