Understanding Middlewares in NestJS - A Basic Guide

- Published on
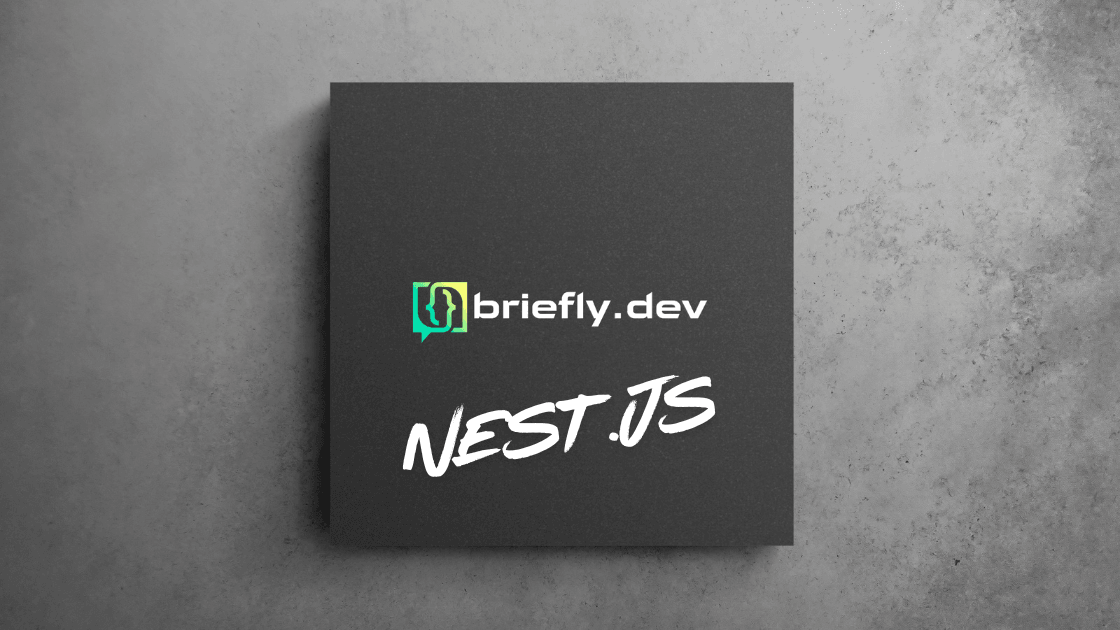
Middlewares in NestJS function like interceptors in that they process incoming requests before they reach the route handlers. They provide an efficient way to handle tasks like logging, validation, or security, allowing for code reuse and clean separation of concerns.
In this post, we'll explain what middlewares are and how to create a basic middleware that logs requests.
What Are Middlewares in NestJS?
In NestJS, a middleware is a function that is called before the route handler is invoked. Middlewares can:
- Log requests
- Authenticate users
- Validate requests
- Add headers or modify requests/responses
They have access to the request and response objects and can modify them if needed. Middlewares can be applied to specific routes, controllers, or globally.
Creating a Basic Middleware in NestJS
Let’s create a simple middleware that logs each incoming request to the console.
- Create a New Middleware: In NestJS, you can generate a middleware using the CLI:
nest g middleware logger
This will generate a new middleware file. Here's what it could look like:
import { Injectable, NestMiddleware } from '@nestjs/common'
import { Request, Response, NextFunction } from 'express'
@Injectable()
export class LoggerMiddleware implements NestMiddleware {
use(req: Request, res: Response, next: NextFunction) {
console.log(`Request... Method: ${req.method}, URL: ${req.url}`)
next()
}
}
The LoggerMiddleware simply logs the HTTP method and URL of each incoming request. The next() function ensures that the request continues its journey to the route handler.
- Apply the Middleware: You can apply the middleware at different levels in NestJS. To apply it globally, go to your main.ts file and modify it like this:
import { NestFactory } from '@nestjs/core'
import { AppModule } from './app.module'
import { LoggerMiddleware } from './logger.middleware'
async function bootstrap() {
const app = await NestFactory.create(AppModule)
app.use(new LoggerMiddleware().use)
await app.listen(3000)
}
bootstrap()
This will log every incoming request to your server.