Data Types in JavaScript

- Published on
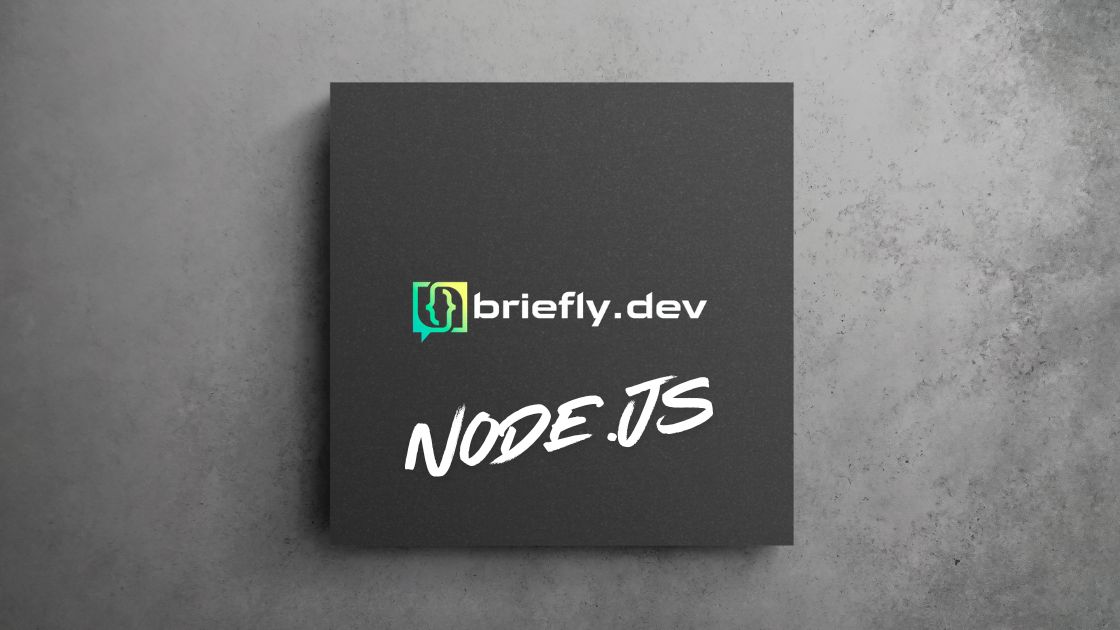
JavaScript, as a dynamically typed language, offers various data types to handle different kinds of data. From primitive types like strings and numbers to more complex types like objects and arrays.
Primitive Data Types
- String
Used for representing textual data. It's a sequence of characters enclosed in single quotes, double quotes, or backticks (for template literals).
let greeting = 'Hello, world!';
- Number
Represents both integer and floating-point numbers. JavaScript has one number type and handles decimals and integers the same way.
let integer = 10;
let float = 10.5;
- Boolean
Represents a logical entity and can have two values: true and false.
let isJavaScriptFun = true;
- Undefined
A variable that has not been assigned, it has the value = undefined.
let thisVariableIsUndefined;
- Null
Represents a deliberate non-value. It is intended to represent an empty value for a variable.
let result = null;
- Symbol
Introduced in ES6, symbols are unique and immutable data types and may be used as an identifier for object properties.
let sym = Symbol('description_here');
Complex Data Types
- Object
Objects are collections of properties, where each property is a key-value pair. Objects are used to store various keyed collections and more complex entities.
let person = {
name: "John",
age: 30
};
- Array
Arrays are a type of object used for storing multiple values in a single variable. Each item in an array has a numeric position, known as its index.
let colors = ['Red', 'Green', 'Blue'];
Special Data Types
- Function
Functions are procedural data types, which means they can be executed. They are objects, allowing them to have properties and be passed around and manipulated like any other object.
function greet(name) {
return `Hello, ${name}!`;
}
Stay tuned for more JavaScript tutorials and tips. Happy coding!