Exploring the CONSOLE Object in JavaScript

- Published on
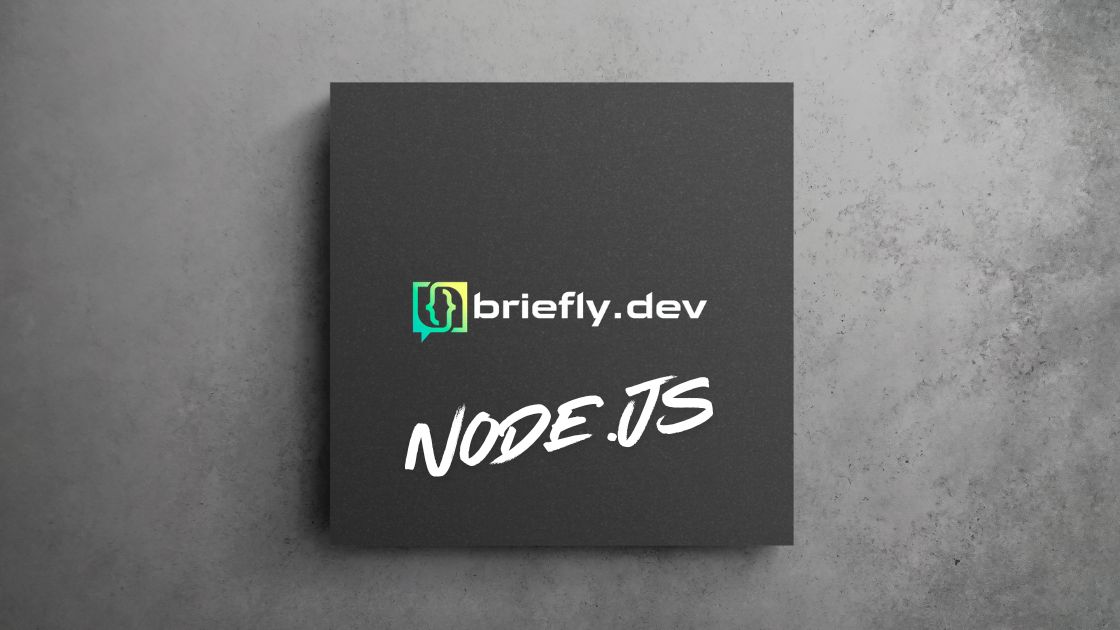
The console object in JavaScript is a powerful tool for debugging and logging information. It's not just about console.log — there are many methods available that can help to understand or show what's happening in the code. Let's explore some of the most useful console methods.
Basic Logging with console.log
The most commonly used method is console.log, which outputs messages to the console. It's great for basic debugging and logging.
console.log('Hello, world!');
Warning and Error Messages
Use console.warn and console.error to display warnings and error messages.
console.warn('This is a warning!');
console.error('This is an error!');
For general information that isn't as critical as a warning or error, use console.info.
console.info('This is an informational message.');
Displaying Objects and Arrays with console.table
The console.table method is handy for displaying objects and arrays in a tabular format, making it easier to read complex data structures.
const users = [
{ name: 'Alice', age: 25 },
{ name: 'Bob', age: 30 },
{ name: 'Charlie', age: 35 }
];
console.table(users);
Grouping Messages with console.group and console.groupEnd
Group related messages together for better organization using console.group and console.groupEnd.
console.group('User Details');
console.log('Name: Alice');
console.log('Age: 25');
console.groupEnd();
Timing with console.time and console.timeEnd
Measure the time taken by a piece of code using console.time and console.timeEnd.
console.time('Array initialization');
const arr = new Array(1000000).fill(0);
console.timeEnd('Array initialization');
Clearing the Console with console.clear
Clear all messages from the console with console.clear.
console.clear();