Hoisting in JavaScript

- Published on
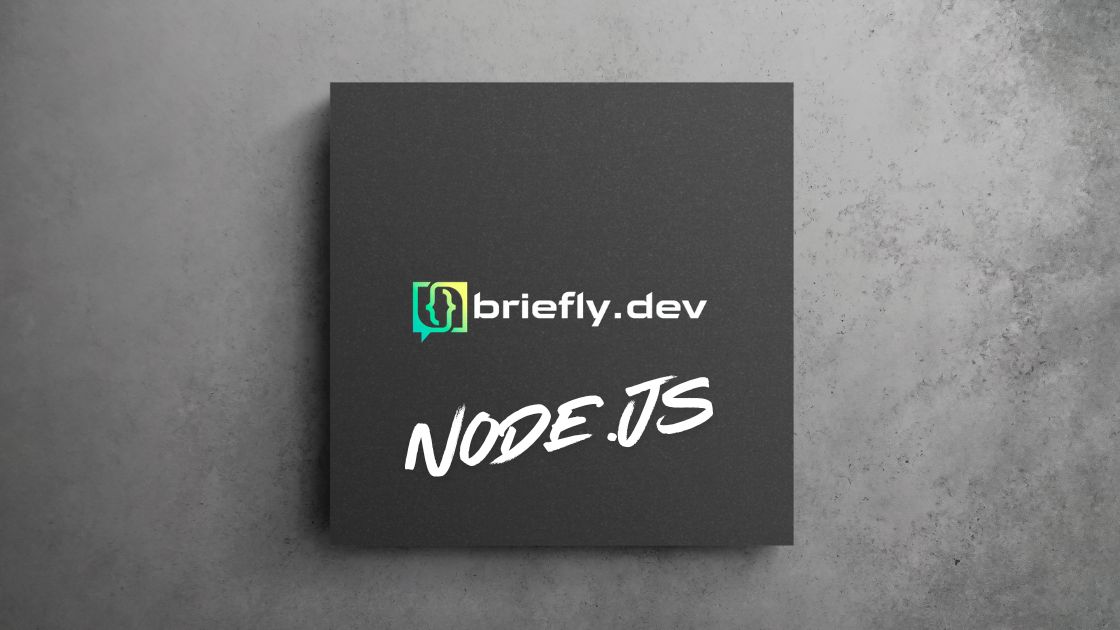
Have you ever wondered why you can access a variable or invoke a function that is defined later in your code? In JavaScript, hoisting is a behavior that moves variable and function declarations to the top of their containing scope during the compilation phase, before the code has been executed. Let’s explore how it works and how you can avoid common pitfalls associated with it.
Types of Hoisting
Variable Hoisting**
In JavaScript, variables declared with var are hoisted to the top of their scope and initialized with a value of undefined. Let’s look at an example:
console.log(myVar); // undefined
var myVar = 'Hello, world!';
In this case, myVar is hoisted to the top of its scope. When console.log is called, myVar exists but its initialization with 'Hello, world!' has not yet occurred, so it logs undefined.
Variables declared with let and const are also hoisted but not initialized, leading to a Reference Error if they are accessed before being declared:
console.log(myLet); // ReferenceError: Cannot access 'myLet' before initialization
let myLet = 'Hello, world!';
Function Hoisting
Functions that are declared as function declarations are hoisted to the top of their scope and are fully defined:
hello(); // Outputs: "Hello, world!"
function hello() {
console.log("Hello, world!");
};
However, function expressions are treated like variable hoisting. The variable declaration is hoisted, but the function definition is not hoisted:
hello(); // ReferenceError: Cannot access 'hello' before initialization
const hello = function() {
console.log("Hello, world!");
};
Best Practices to Avoid Hoisting Issues
- Use let and const - To avoid issues with variable hoisting, prefer let and const over var for variable declarations.
- Declare Functions Early - Declare functions at the top of their scope if they are needed before their declaration point in the code.
- Initialize Variables Before Use - Always initialize variables before you use them to avoid undefined values.