How JavaScript Works - A Deep Dive into the JavaScript Engine

- Published on
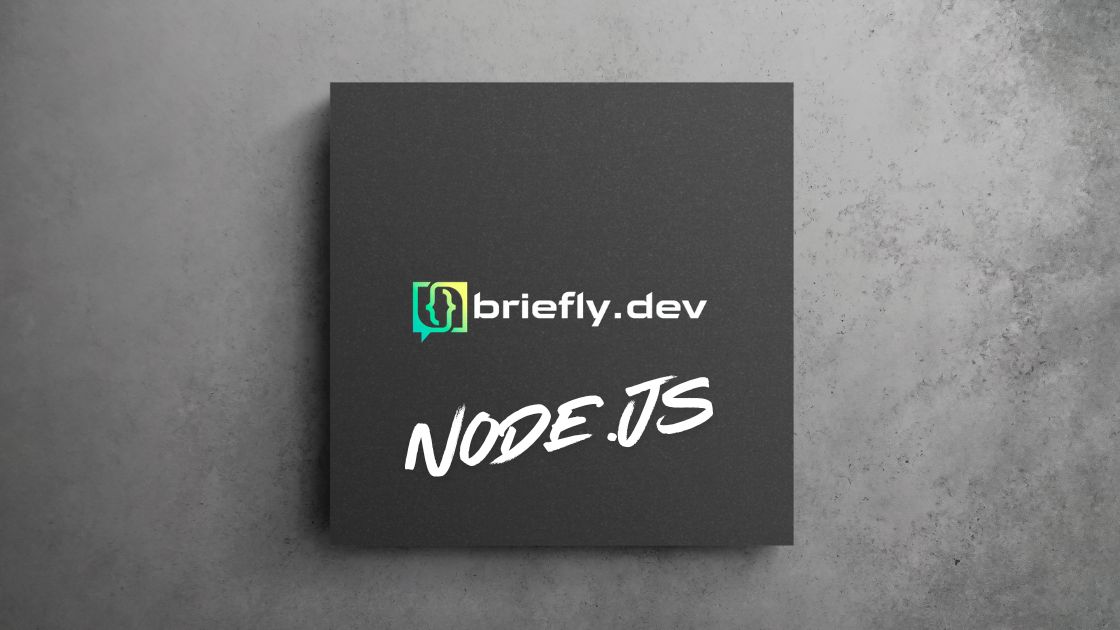
We know JavaScript, but how does JavaScript work behind the scenes? Understanding it can help write more efficient and optimized code.
The JavaScript Engine
A JavaScript engine executes JavaScript code. Some of the most well-known JavaScript engines include Google’s V8 (used in Chrome and Node.js), Mozilla’s SpiderMonkey (used in Firefox), and Microsoft’s Chakra (used in Edge).
Key Components of the JavaScript Engine
Parser:
Reads the JavaScript code and converts it into an Abstract Syntax Tree (AST). Syntax errors are detected at this stage.
Interpreter:
Executes the AST directly or generates bytecode to be executed by the runtime. In the case of a Just-In-Time (JIT) compiler, it interprets the code and identifies "hot" code paths for optimization.
JIT Compiler:
Compiles hot code paths into optimized machine code at runtime. Improves performance by applying various optimizations.
Garbage Collector:
Manages memory allocation and deallocation. Automatically frees up memory that is no longer in use by the program.
How JavaScript Works: From Code to Execution
- Parsing
When a JavaScript engine receives JavaScript code, the first step is parsing. The parser reads the code and checks for syntax errors. If the code is valid, it is converted into an Abstract Syntax Tree (AST), which represents the structure of the code in a tree format.
let x = 10;
console.log(x);
The AST for this code might look something like this:
VariableDeclaration, VariableDeclarator, Identifier (x), Literal (10), ExpressionStatement, CallExpression, MemberExpression, Identifier (console), Identifier (log), Identifier (x)
- Compilation
In modern JavaScript engines, after the AST is generated, the code is compiled into bytecode. The interpreter executes this bytecode. In engines like V8, a JIT compiler is used to compile frequently executed code into optimized machine code during execution, which significantly boosts performance.
- Execution
The JavaScript engine executes the compiled bytecode or machine code. During execution, the engine manages various tasks such as:
- Scope Management: Keeping track of variables and their scopes.
- Memory Management: Allocating and freeing memory as needed.
- Garbage Collection: Automatically cleaning up unused memory to prevent memory leaks.
Event Loop and Concurrency
JavaScript is single-threaded, meaning it executes one task at a time. However, it is capable of handling asynchronous operations using the event loop.
Call Stack:
Keeps track of function calls and execution contexts.
Web APIs:
Provides asynchronous features like setTimeout, fetch, and DOM events.
Callback Queue:
Stores callback functions to be executed when the call stack is empty.
Event Loop:
Continuously checks the call stack and callback queue, pushing callbacks onto the call stack when it is empty.
Example: Asynchronous Execution
console.log('Start');
setTimeout(() => {
console.log('Timeout');
}, 1000);
console.log('End');
Execution flow:
- console.log('Start') is executed and printed to the console.
- setTimeout is called and the callback is registered in the Web API.
- console.log('End') is executed and printed to the console.
- After 1000ms, the callback from setTimeout is pushed to the callback queue.
- The event loop moves the callback to the call stack once it is empty, and console.log('Timeout') is executed.