Password Hashing in Express.js with Bcrypt
By Łukasz Kallas

- Published on
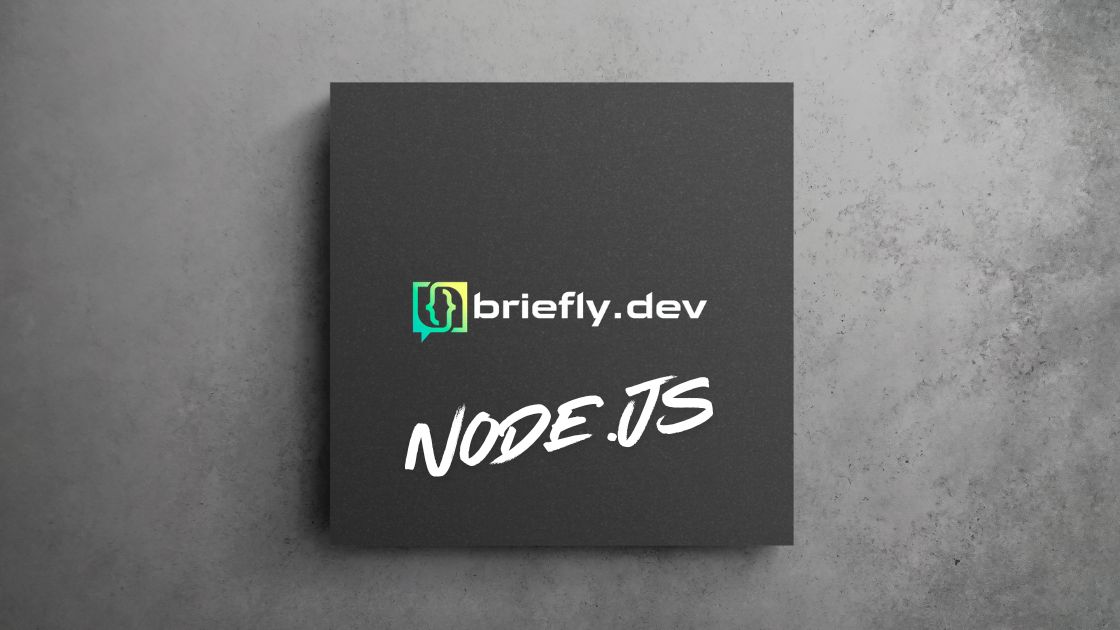
Sharing
Today, we'll go through the basics of integrating Bcrypt (popular library for password hashing in Node.js) into an Express.js application using an array to store users instead of a database. Additionally, we'll include a hands-on guide on how to install Bcrypt, create users, and authenticate using Postman.
Sample code:
const express = require('express');
const bcrypt = require('bcrypt');
const app = express();
app.use(express.json());
const PORT = 8000;
const users = [];
app.post('/register', (req, res) => {
const { username, password } = req.body;
const salt = bcrypt.genSaltSync(10);
const passwordSalted = bcrypt.hashSync(password, salt);
users.push({ username, password: passwordSalted });
res.status(201).send('User registered');
});
app.post('/login', (req, res) => {
const { username, password } = req.body;
const user = users.find((user) => user.username === username);
if (user && bcrypt.compareSync(password, user.password)) {
res.send('User logged in');
} else {
res.status(401).send('Invalid credentials');
}
});
app.listen(PORT, () => console.log(`Server running on port ${PORT}`));