Understanding JavaScript Module Types
By Łukasz Kallas

- Published on
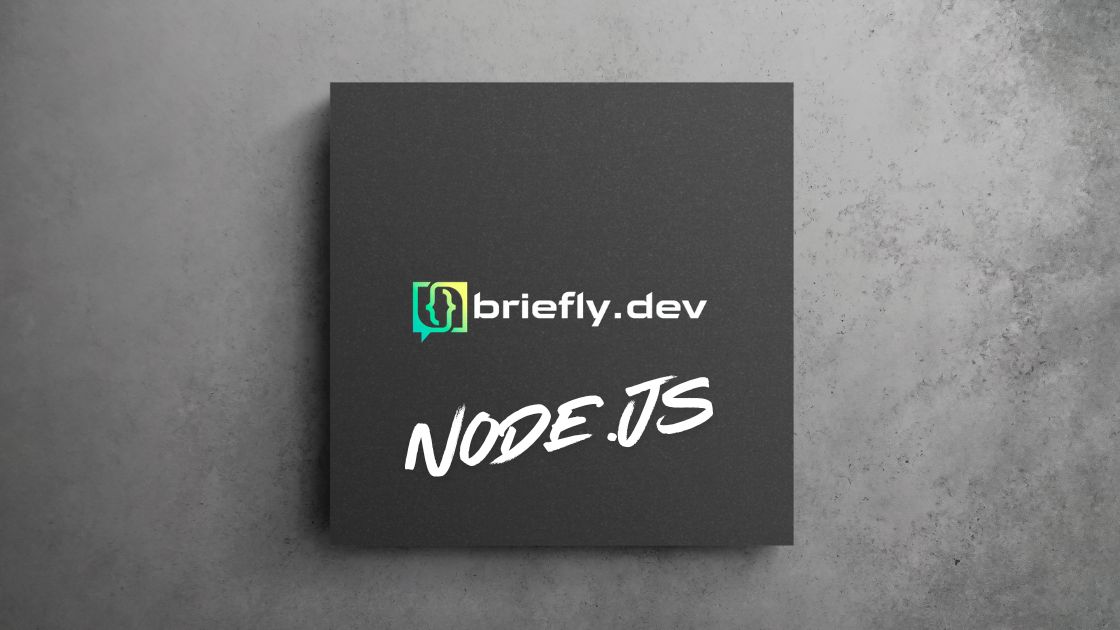
Sharing
JavaScript modules are a way to organize and manage code by encapsulating functionality within reusable, self-contained units. There are three primary module systems: CommonJS, AMD, and ES6 modules.
CommonJS Modules
CommonJS is a module system used primarily in Node.js. It allows you to export and import modules using module.exports and require().
Characteristics:
- Synchronous loading.
- Suitable for server-side development.
- Each file is treated as a separate module.
Example:
module.js:
const sayHello = () => {
console.log('Hello, world!');
};
module.exports = sayHello;
main.js:
const sayHello = require('./module');
sayHello(); // Outputs: Hello, world!
AMD (Asynchronous Module Definition)
AMD is a module system designed for asynchronous loading of modules in the browser. It uses the define function to define modules and require to import them.
Characteristics:
- Asynchronous loading.
- Suitable for client-side development.
- Often used with RequireJS.
Example:
module.js:
define([], function() {
return {
sayHello: function() {
console.log('Hello, world!');
}
};
});
main.js:
require(['module'], function(module) {
module.sayHello(); // Outputs: Hello, world!
});
ES6 Modules (ECMAScript 2015)
ES6 modules are the standard module system in JavaScript, supported natively by modern browsers and Node.js. They use the import and export syntax.
Characteristics:
- Synchronous and asynchronous loading.
- Suitable for both client-side and server-side development.
- Supports static analysis and tree shaking.
Example:
module.js:
export const sayHello = () => {
console.log('Hello, world!');
};
main.js:
import { sayHello } from './module.js';
sayHello(); // Outputs: Hello, world!
Comparison and Use Cases
CommonJS:
- Use When: Working with Node.js or server-side JavaScript.
- Advantages: Synchronous loading, simplicity.
- Disadvantages: Not suitable for the browser without bundling.
AMD:
- Use When: Developing browser-based applications that require asynchronous loading.
- Advantages: Asynchronous loading, good for large-scale applications.
- Disadvantages: More complex syntax compared to CommonJS and ES6 modules.
ES6 Modules:
- Use When: Writing modern JavaScript for both client-side and server-side applications.
- Advantages: Native support in modern environments, better tooling and optimization.
- Disadvantages: Requires transpilation for older environments that do not support ES6 modules.