Understanding JavaScript Proxy
By Łukasz Kallas

- Published on
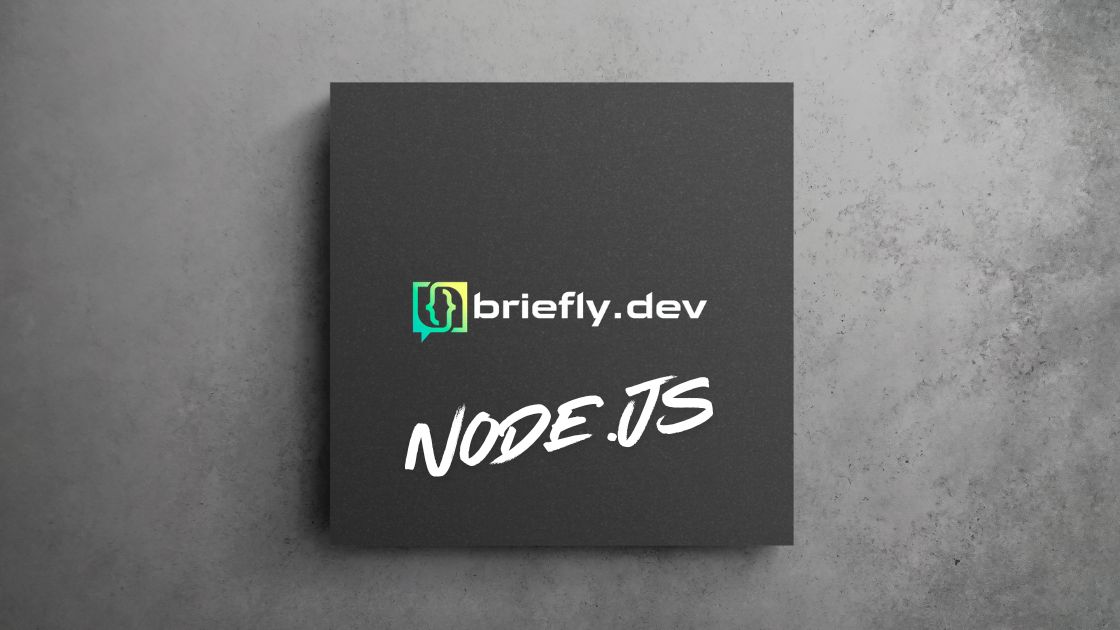
Sharing
JavaScript provides powerful tools for developers to customize the behavior of objects. One such tool is the Proxy object.
What is a JavaScript Proxy?
A Proxy object enables you to define custom behavior for fundamental operations (e.g., property lookup, assignment, enumeration, function invocation, etc.). It wraps an object and intercepts operations performed on it, allowing you to manipulate these operations as needed.
Basic Syntax
A Proxy is created using the Proxy constructor, which takes two arguments:
- target - The object that the proxy virtualizes.
- handler - An object that defines which operations will be intercepted and how to redefine them.
const proxy = new Proxy(target, handler);
Common Use Cases
- Validation - Ensuring that certain conditions are met when interacting with an object.
- Property Access Control - Controlling how properties are accessed or modified.
- Logging/Monitoring - Logging operations performed on an object for debugging or monitoring purposes.
Example: Basic Proxy
const target = {
message1: "hello",
message2: "world"
};
const handler = {
get: function(obj, prop) {
console.log(`Property ${prop} has been accessed.`);
return obj[prop];
}
};
const proxy = new Proxy(target, handler);
console.log(proxy.message1);
// Logs: Property message1 has been accessed.
// Returns: hello
Interceptable Operations
The handler object can define various traps, each corresponding to a fundamental operation:
- get(target, property, receiver): Traps reading a property.
- set(target, property, value, receiver): Traps setting a property value.
- has(target, property): Traps the in operator.
- deleteProper(target, property): Traps delete.
- apply(target, thisArg, argumentsList): Traps a function call.
- construct(target, argumentsList, newTarget): Traps the new operator.
Below is the YouTube hands-on solving Codewars Kata using Proxy: