KISS Principle in Software Development

- Published on
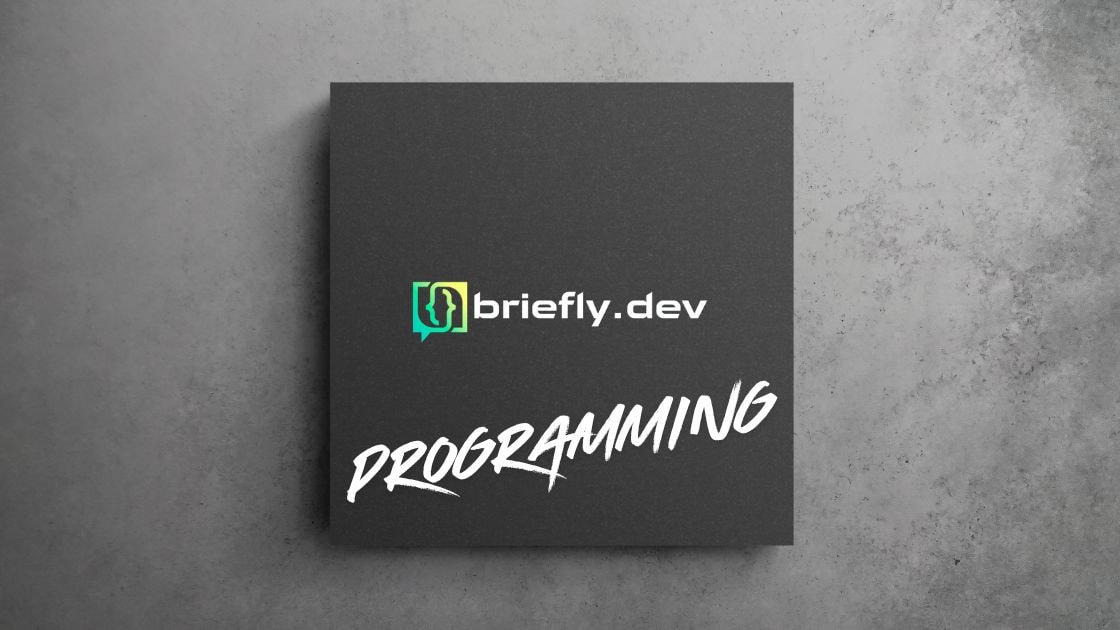
In the world of software development, complexity can often be the enemy of success.
What is the KISS Principle?
The KISS principle emphasizes simplicity in design and implementation. The idea is to avoid unnecessary complexity by keeping systems as simple as possible.
Why is Simplicity Important?
Easier Maintenance:
Simple code is easier to read, understand, and maintain. Developers can quickly grasp the functionality and make changes without introducing bugs.
Improved Debugging:
With fewer moving parts, simple systems are easier to debug. Problems can be identified and resolved faster.
Better Performance:
Simple solutions often perform better because they avoid the overhead associated with complex implementations.
Enhanced Collaboration:
When code is straightforward, team members can collaborate more effectively. New developers can get up to speed quickly, and code reviews become more productive.
Applying the KISS Principle
- Write Clear and Concise Code
Aim to write code that is easy to understand. Use meaningful variable names, avoid overly complex logic, and adhere to coding standards and conventions.
// Complex
function calc(a, b) {
if (b === 0) {
return 0;
} else {
return a / b;
}
}
// Simple
function divide(a, b) {
if (b === 0) return 0;
return a / b;
}
- Avoid Over-Engineering
Resist the temptation to add features or complexity that are not necessary. Focus on solving the problem at hand with the simplest solution possible.
// Over-Engineered
class Calculator {
constructor() {
this.result = 0;
}
add(a, b) {
this.result = a + b;
return this.result;
}
subtract(a, b) {
this.result = a - b;
return this.result;
}
}
// Simple
function add(a, b) {
return a + b;
}
function subtract(a, b) {
return a - b;
}
- Refactor Regularly
Regularly review and refactor your code to eliminate unnecessary complexity. Simplifying existing code can lead to better performance and maintainability.
- Use Libraries and Frameworks Wisely
Leverage existing libraries and frameworks to simplify your codebase. However, avoid over-reliance on them if it adds unnecessary complexity.
- Embrace Modular Design
Break down your code into smaller, reusable modules. This approach not only simplifies individual components but also makes your overall system easier to understand and manage.