Regular Expressions in Programming
By Łukasz Kallas

- Published on
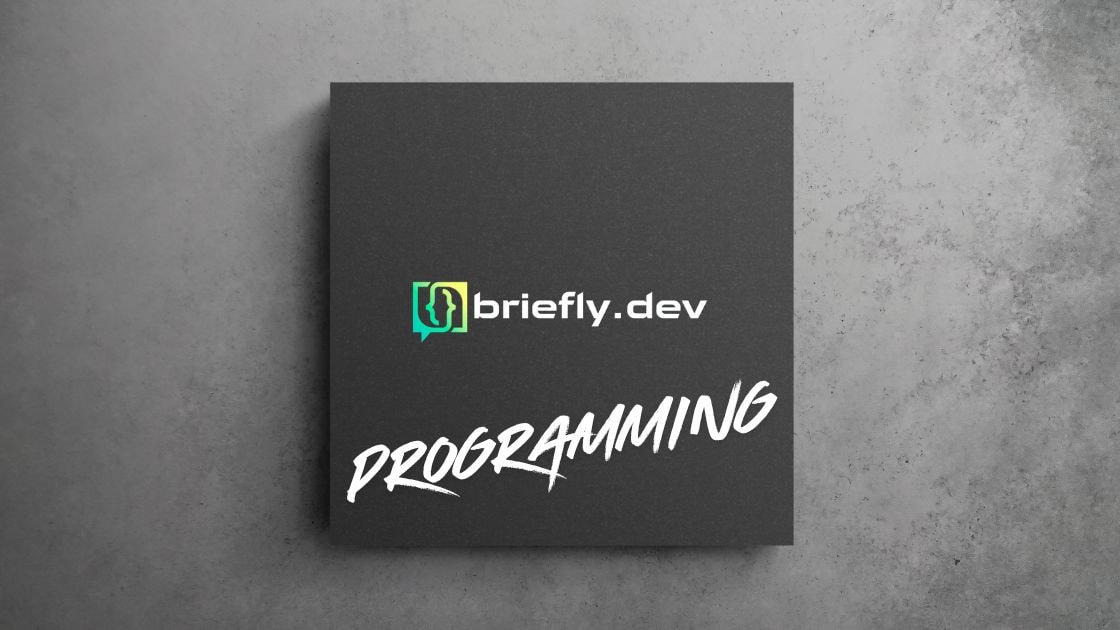
Sharing
Regular expressions, commonly known as regex, are a powerful tool for searching, manipulating, and validating text. They allow developers to create flexible patterns that can match various string formats, making them indispensable for parsing large text files, validating user input, or processing logs. This post introduces regular expressions, how they work, and provides practical examples to demonstrate their utility.
Core Components of Regex
- Literals - Regular characters that match themselves.
- Metacharacters - Characters with special meanings, like . (matches any single character), ^ (matches the start of a string), $ (matches the end of a string).
- Character Classes - Sets of characters that can occur in an input string, e.g., [a-z] matches any lowercase letter.
- Quantifiers - Indicate numbers of characters or expressions to match, e.g., * (zero or more), + (one or more),
{n}
(exactly n times).
Common Regex Operations
- Search - Find patterns within a text.
- Match - Check if the entire string matches a pattern.
- Replace - Substitute parts of the text based on regex patterns.
- Split - Break a string into pieces at each match.
Practical Examples
- Validating Email (This pattern ensures that an email address consists of permitted characters, includes an @ symbol, followed by a domain.)
^[a-zA-Z0-9_.+-]+@[a-zA-Z0-9-]+\.[a-zA-Z0-9-.]+$
- Extracting Dates (Matches dates in formats like dd-mm-yyyy, d/m/yy.)
\b\d{1,2}[-/]\d{1,2}[-/]\d{2,4}\b