UUID - Universally Unique Identifiers

- Published on
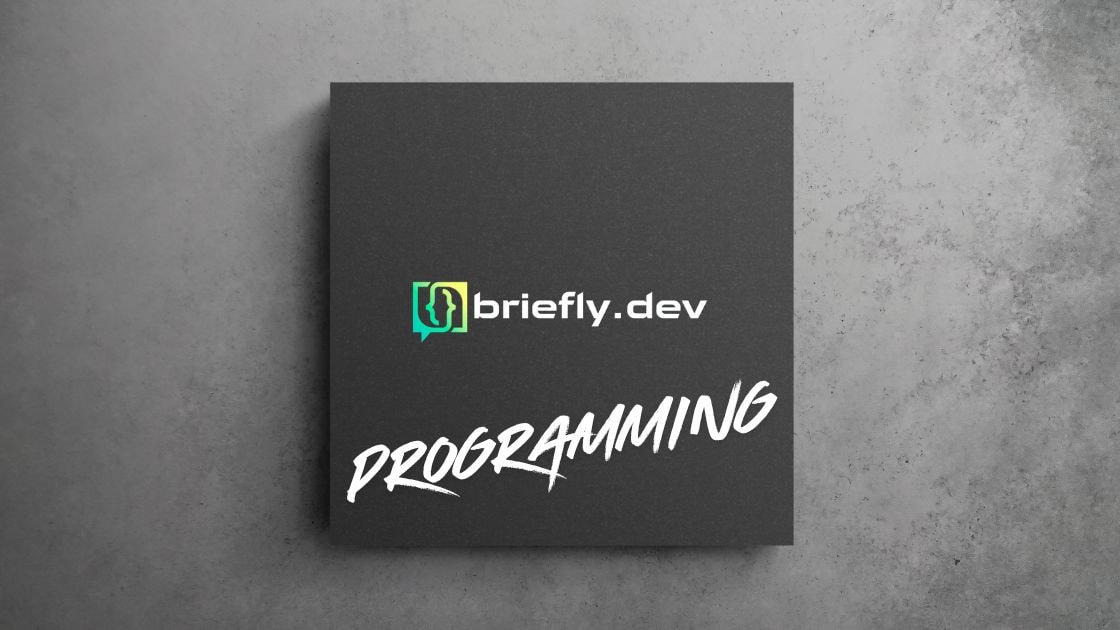
In software development, generating unique identifiers is a common requirement.
What is a UUID?
A UUID (Universally Unique Identifier) is a 128-bit number used to uniquely identify information in computer systems.
Structure of a UUID
A typical UUID is represented as a 36-character string, containing 32 hexadecimal digits, displayed in five groups separated by hyphens in the form 8-4-4-4-12.
Example: 550e8400-e29b-41d4-a716-446655440000
Generating UUIDs
UUID Version 1 (Timestamp-Based)
Version 1 UUIDs use the current timestamp and the machine's MAC address to generate a unique identifier. This ensures uniqueness but can potentially expose the generation time and the machine's identity.
UUID Version 4 (Random-Based)
Version 4 UUIDs are generated using random or pseudo-random numbers. This method provides a high level of uniqueness without revealing any identifiable information about the machine or the time of generation.
Importance of UUIDs
Global Uniqueness:
UUIDs are guaranteed to be unique across all systems, making them ideal for distributed systems where unique identification is crucial.
Scalability:
Because UUIDs are unique and can be generated independently, they are perfect for applications that need to scale across multiple servers and databases.
Data Merging:
UUIDs are useful when merging data from different sources, ensuring that there are no conflicts due to duplicate identifiers.
Database Keys:
Using UUIDs as primary keys in databases can simplify data replication and synchronization across distributed systems.
How to Generate UUIDs
UUIDs can be generated using various programming languages and libraries. Here are a few examples:
Using Python
import uuid
# Generate a UUID version 1
uuid1 = uuid.uuid1()
print(uuid1)
# Generate a UUID version 4
uuid4 = uuid.uuid4()
print(uuid4)
Using JavaScript
const { v1: uuidv1, v4: uuidv4 } = require('uuid');
// Generate a UUID version 1
console.log(uuidv1());
// Generate a UUID version 4
console.log(uuidv4());