A Guide to useState Hook in React

- Published on
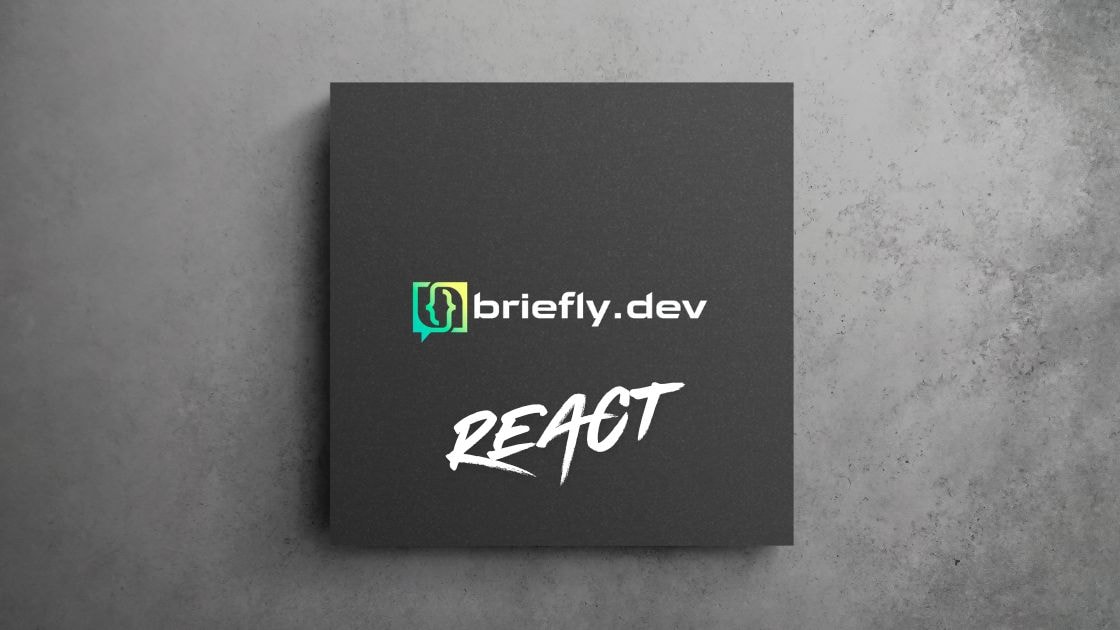
The useState hook is one of the most fundamental hooks in React, allowing you to add state to your functional components. We'll explore the basics of useState and dive into some advanced concepts. There is also a hands-on YouTube tutorial available to guide you through practical examples.
Basic Usage of useState
The useState hook allows you to create state variables in functional components. Here's a simple example:
import React, { useState } from 'react';
export default function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<h1>Counter</h1>
<p>{count}</p>
<button onClick={() => setCount(count + 1)}>
Increment
</button>
</div>
);
}
- useState(0) initializes the count state variable to 0.
- setCount is a function that updates the value of count.
- Clicking the button increases the count value by 1.
Initial State
The argument passed to useState is the initial state. It can be a primitive value, an object, or any data type. You can even use a function to set the initial state if the computation is expensive:
const [items, setItems] = useState(() => {
const initialItems = loadInitialItems();
return initialItems;
});
Updating State
The setState function returned by useState can accept either a new state value or a function that takes the current state and returns a new state. This is particularly useful when the new state depends on the previous state:
setCount(prevCount => prevCount + 1);
Using the functional form of setState ensures that you're working with the most recent state, especially in asynchronous operations.
Multiple State Variables
You can use multiple useState hooks to manage different pieces of state. Each call to useState creates an independent piece of state:
const [count, setCount] = useState(0);
const [name, setName] = useState('');
This allows you to organize and manage state more effectively in your components.
State Persistence
It's important to note that state created with useState is local to the component. When the component re-renders, the state persists. However, if the component is unmounted, the state is lost. To persist state across re-renders or component unmounting, consider using other mechanisms like global state or local storage.