Understanding the useContext Hook in React
By Ćukasz Kallas

- Published on
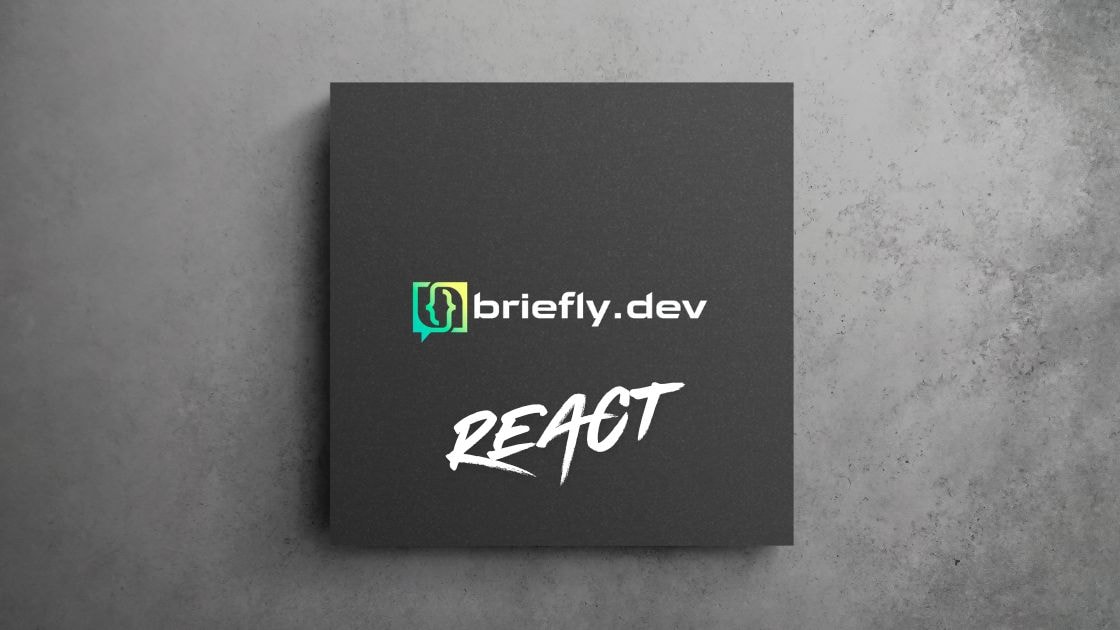
Sharing
In React, state management across components can sometimes become tricky, especially when you have to pass data down through multiple layers of components. The useContext hook is a solution to this problem, allowing components to subscribe to React context without the need for deeply nested props.
When to Use useContext
- Global State: When you have global data that needs to be accessed by many components (e.g., user authentication, theme settings).
- Avoiding Prop Drilling: When passing data through many layers of components would be cumbersome.
- Cross-Component Communication: When multiple components need to react to the same state change.
How to Use useContext
- Create a Context: First, you create a context using createContext().
export const ThemeContext = createContext()
- Provide Context Value: Wrap your component tree with the context provider and pass the value you want to share.
export default function ThemeProvider({ children }) {
const [theme, setTheme] = useState('light')
return <ThemeContext.Provider value={{ theme, setTheme }}>{children}</ThemeContext.Provider>
}
- Wrap Your App with the Provider
import ThemeProvider from '@/context/theme.context'
export default function App({ Component, pageProps }) {
return (
<ThemeProvider>
<Component {...pageProps} />
</ThemeProvider>
)
}
- Consume Context Value: Use the useContext hook to access the context value in your components.
import { useContext } from 'react'
import { ThemeContext } from '../context/theme.context'
export default function Home() {
const { theme, setTheme } = useContext(ThemeContext)
return (
<div
style={{
backgroundColor: theme === 'light' ? 'white' : 'black',
color: theme === 'light' ? 'black' : 'white',
}}
>
<h1>Home</h1>
<p>Current theme is {theme}</p>
<button onClick={() => setTheme(theme === 'light' ? 'dark' : 'light')}>Toggle Theme</button>
</div>
)
}