Optimization with useMemo Hook in React
By Łukasz Kallas

- Published on
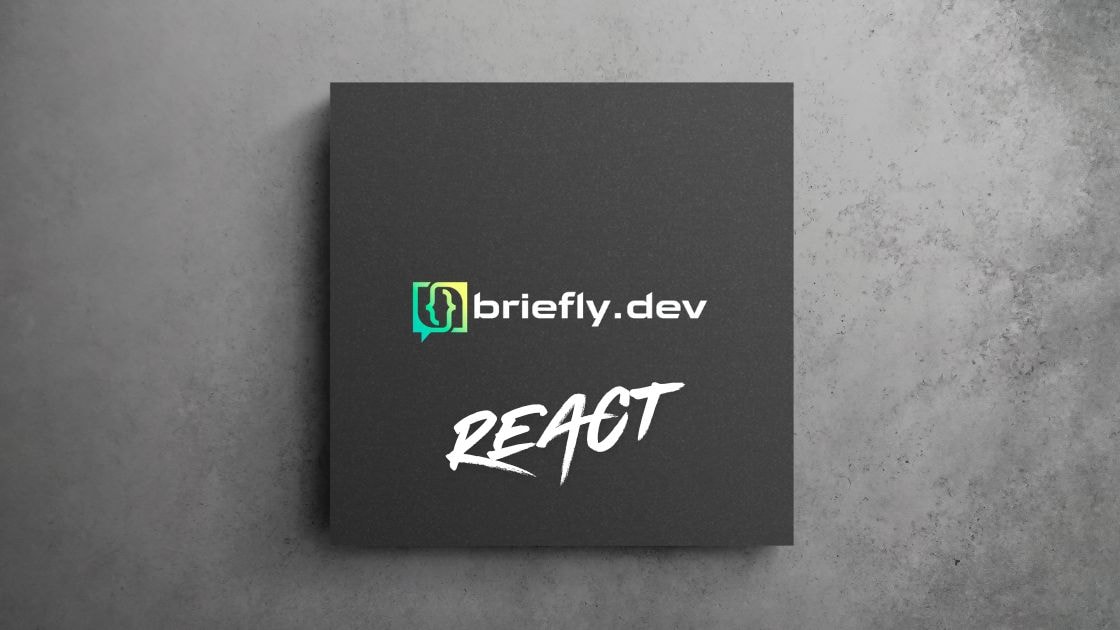
Sharing
What is useMemo?
The useMemo hook allows you to memoize the result of a function so that it's only recalculated when its dependencies change. This is particularly useful for expensive computations that don't need to run on every render.
const expensiveFunction = () => {
let result = 0
for (let i = 0; i < 1000000000; i++) {
result += i
}
return result
}
const memoExpensiveFunction = useMemo(() => expensiveFunction(), [a, b])
The expensiveCalculation function is only recalculated when a or b changes.
When to Use useMemo
- Expensive Calculations: When you have computations that take significant time.
- Referential Equality: To prevent unnecessary re-renders in child components by ensuring that props maintain the same reference unless dependencies change.
Avoiding Overuse
While useMemo can optimize performance, overusing it can lead to unnecessary complexity. It's essential to measure performance and identify bottlenecks before introducing memoization.
Common Pitfalls
- Ignoring Dependencies: Always include all dependencies in the dependency array to avoid bugs.
- Overhead: Memoization itself has a cost. If the computation is trivial, memoization might not provide benefits.
- Stale Closures: Be aware of variables used within the memoized function. If they change, ensure they're included in dependencies.