Understanding the useEffect Hook in React - From Basics to Advanced Concepts

- Published on
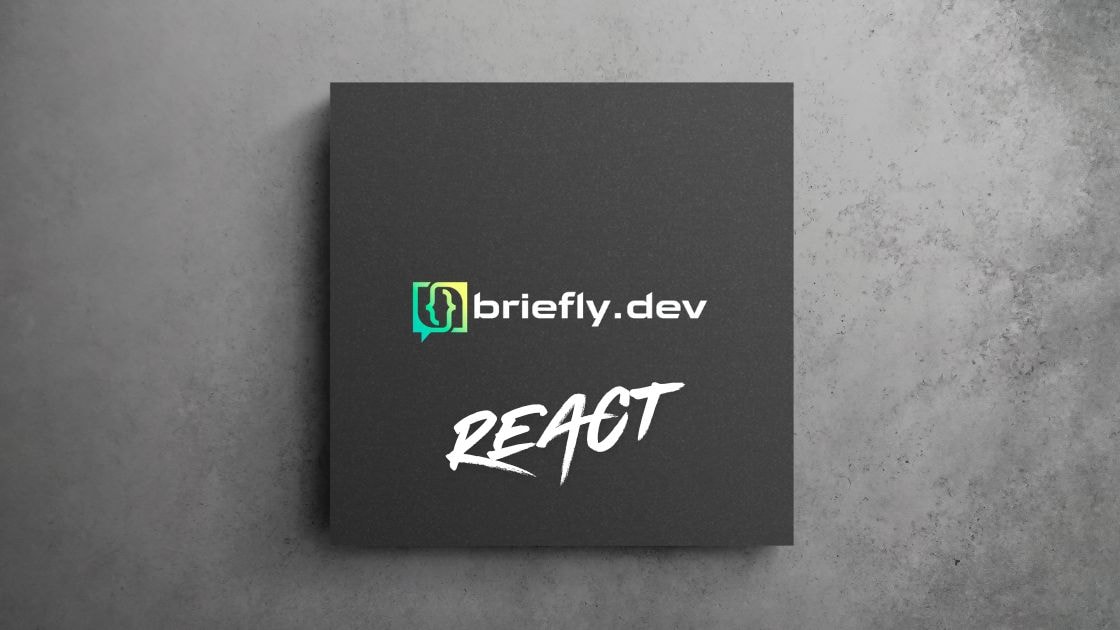
React's useEffect hook is one of the most commonly used hooks in functional components. It allows to perform side effects in components, such as fetching data, updating the DOM, setting up subscriptions, and more.
What is useEffect?
The useEffect hook lets you perform side effects in your React components. It's a replacement for lifecycle methods like componentDidMount, componentDidUpdate, and componentWillUnmount in class-based components.
Basic Syntax
useEffect(() => {
console.log('useEffect called');
});
This will run the side effect after every render, including the initial render.
The Dependency Array
To control when the effect runs, you can provide a dependency array as the second argument to useEffect:
useEffect(() => {
console.log('useEffect called');
}, [dependencies]);
- If you pass an empty array [], the effect will only run once, after the initial render.
- If you pass specific dependencies [dep1, dep2], the effect will run only when those dependencies change.
Example: Fetching Data
const fetchDummyData = async () => new Promise((resolve) => {
setTimeout(() => {
resolve('dummy data');
}, 2000);
});
useEffect(() => {
console.log('useEffect called');
fetchDummyData().then((data) => {
console.log('data', data);
});
},[]);
Cleanup Function
If your effect involves setting up subscriptions or timers, you'll want to clean up those resources when the component unmounts or before the effect runs again. You can return a cleanup function from useEffect:
useEffect(() => {
console.log('useEffect called');
const timer = setTimeout(() => {
console.log('timer called');
}, 5000);
return () => {
clearTimeout(timer);
}
},[]);